Programowanie w systemie UNIX/C
Wygląd
Specjalistyczny podręcznik Programowania w C
Wstęp
[edytuj]obiektowe-c
[edytuj]Objective-C – rozszerzenie języka C o możliwości obiektowe, wzorowane na Smalltalku. Objective-C przyjął drogę całkowicie odmienną od C++. Jest używany głównie w frameworku Cocoa w systemie OS X oraz w iOS.
kompilacja na Ubuntu [1]
Programowanie równoległe
[edytuj]- CPU
- MIC = CPU z wieloma rdzeniami (SIMD, OpenMP) i koprocesor Xeon Phi
- pthreads = wątki
- GNU UPC
- ispc - Intel SPMD Program Compiler. An open-source compiler for high-performance SIMD programming on the CPU
- Procesor Cell i Sony PS3
- GPU
Biblioteki, moduły i pakiety
[edytuj]- stb = stb single-file public domain libraries for C/C++ by Sean Barrett
- par = single-file C libraries from Philip Allan Rideout
- Linuxconfig.org: c-development-on-linux-packaging-for-debian-and-fedora-xi
- libasan = the address sanitizer for gcc and clang [2]
Interfejs programistyczny aplikacji (ang. Application Programming Interface, API)
[edytuj]- standardowe (libc, ...)
- pthreads
- glib wykorzystywane w GTk i Gnome
Standardy
[edytuj]- POSIX
Wskazówki POSIX dotyczące składni poleceń
numeryczne
[edytuj]- imath is a C++ and python library of 2D and 3D vector, matrix, and math operations for computer graphics
- GSL
- PARI (libpari)
- Anant -- Algorithmic 'n Analytic Number Theory by Linas Vepstas
- The multiple-digit approach
- the multiple-component approach
- doubledouble by Keith Briggs
- quad_float in Victor Shoup's NTL library.
- []
- qd
- GQD = qd na GPU
- GPU
- przenośne (ang. portable) = które nie wymagają instalacji:
- APR
"The multiple-digit approach can representamuch larger range of numbers, whereas the multiple-component approach has the advantage in speed."
Grafika
[edytuj]- Grafika[3][4]
- OpenGL oraz Glut, Glew, GLSL ....
- Gegl
- GraphicsGems - Code for the "Graphics Gems" book series
- Code developed for articles in the "Journal of Graphics Tools"
- libgd = GD Graphics (Draw) Library : sudo apt-get install libgd-dev
- OpenCV
- libpng
- cjpeg - CLI program, który konwertuje plik lub stdout do pliku jpg
- Programowanie_w_systemie_UNIX/c_grafika#Plotutils i libplot
- libvips
- openexr
- AOMediaCodec libavif Library for encoding and decoding .avif files ( HDR)
- Gdk-pixbuf Image loading library
- cairo, pixman
- Pango - is a library for laying out and rendering of text, with an emphasis on internationalization.
- GR is a universal framework for cross-platform visualization applications.
- plplot
- Heman is a C library of image utilities for dealing with height maps and other floating-point images.
- dislin s a high-level plotting library for displaying data as curves, polar plots, bar graphs, pie charts, 3D-color plots, surfaces, contours and maps.
- gfx a simple graphics library for X11
- picasso is a high quality vector graphic rendering library. It support path , matrix , gradient , pattern , image and truetype font.
- olive.c is a simple graphics library that does not have any dependencies and renders everything into the given memory pixel by pixel.
- raylib A simple and easy-to-use library to enjoy videogames programming
- color
- GUI
- SDL
- gtk
- Glut
- IUP = Portable User Interface
- SFML = Simple and Fast Multimedia Library
- Nuklear - 1 file
- cefapi A simple example on how to use the C API in Chromium Embedded Framework created by Czarek Tomczak.
- Sokol: Simple STB-style cross-platform libraries for C and C++, written in C by Andre Weissflog ( floooh )
- Sokol GP. Minimal efficient cross platform 2D graphics painter in pure C using modern graphics API through the excellent Sokol GFX library. Sokol GP, or in short SGP, stands for Sokol Graphics Painter by Eduardo Bart edubart
- lcui
- webview
- raygui A simple and easy-to-use immediate-mode gui library
Wczytywanie obrazu z użyciem stb_image[5]
#include <stdint.h>
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
int main() {
int width, height, bpp;
uint8_t* rgb_image = stbi_load("image.png", &width, &height, &bpp, 3);
stbi_image_free(rgb_image);
return 0;
}
Tworzenie obrazu z użyciem stb_image
#include <stdint.h>
#define STB_IMAGE_WRITE_IMPLEMENTATION
#include "stb_image_write.h"
#define CHANNEL_NUM 3
int main() {
int width = 800;
int height = 800;
uint8_t* rgb_image;
rgb_image = malloc(width*height*CHANNEL_NUM);
// Write your code to populate rgb_image here
stbi_write_png("image.png", width, height, CHANNEL_NUM, rgb_image, width*CHANNEL_NUM);
return 0;
}
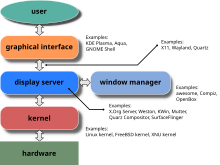
dźwięk
[edytuj]- libsoundio - biblioteka c do obsługi dźwięku
#include <soundio/soundio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
static const float PI = 3.1415926535f;
static float seconds_offset = 0.0f;
static void write_callback(struct SoundIoOutStream *outstream,
int frame_count_min, int frame_count_max)
{
const struct SoundIoChannelLayout *layout = &outstream->layout;
float float_sample_rate = outstream->sample_rate;
float seconds_per_frame = 1.0f / float_sample_rate;
struct SoundIoChannelArea *areas;
int frames_left = frame_count_max;
int err;
while (frames_left > 0) {
int frame_count = frames_left;
if ((err = soundio_outstream_begin_write(outstream, &areas, &frame_count))) {
fprintf(stderr, "%s\n", soundio_strerror(err));
exit(1);
}
if (!frame_count)
break;
float pitch = 440.0f;
float radians_per_second = pitch * 2.0f * PI;
for (int frame = 0; frame < frame_count; frame += 1) {
float sample = sin((seconds_offset + frame * seconds_per_frame) * radians_per_second);
for (int channel = 0; channel < layout->channel_count; channel += 1) {
float *ptr = (float*)(areas[channel].ptr + areas[channel].step * frame);
*ptr = sample;
}
}
seconds_offset = fmod(seconds_offset + seconds_per_frame * frame_count, 1.0);
if ((err = soundio_outstream_end_write(outstream))) {
fprintf(stderr, "%s\n", soundio_strerror(err));
exit(1);
}
frames_left -= frame_count;
}
}
int main(int argc, char **argv) {
int err;
struct SoundIo *soundio = soundio_create();
if (!soundio) {
fprintf(stderr, "out of memory\n");
return 1;
}
if ((err = soundio_connect(soundio))) {
fprintf(stderr, "error connecting: %s\n", soundio_strerror(err));
return 1;
}
soundio_flush_events(soundio);
int default_out_device_index = soundio_default_output_device_index(soundio);
if (default_out_device_index < 0) {
fprintf(stderr, "no output device found\n");
return 1;
}
struct SoundIoDevice *device = soundio_get_output_device(soundio, default_out_device_index);
if (!device) {
fprintf(stderr, "out of memory\n");
return 1;
}
fprintf(stderr, "Output device: %s\n", device->name);
struct SoundIoOutStream *outstream = soundio_outstream_create(device);
if (!outstream) {
fprintf(stderr, "out of memory\n");
return 1;
}
outstream->format = SoundIoFormatFloat32NE;
outstream->write_callback = write_callback;
if ((err = soundio_outstream_open(outstream))) {
fprintf(stderr, "unable to open device: %s", soundio_strerror(err));
return 1;
}
if (outstream->layout_error)
fprintf(stderr, "unable to set channel layout: %s\n", soundio_strerror(outstream->layout_error));
if ((err = soundio_outstream_start(outstream))) {
fprintf(stderr, "unable to start device: %s\n", soundio_strerror(err));
return 1;
}
for (;;)
soundio_wait_events(soundio);
soundio_outstream_destroy(outstream);
soundio_device_unref(device);
soundio_destroy(soundio);
return 0;
}
Debugowanie
[edytuj]- gdb
- backtrace
Dodatkowe materiały
[edytuj]- Programowanie w języku C - Artur Pyszczuk[12]
- Learn C The Hard Way
- c source examples
- Sams Teach Yourself C in 24 Hours by Tony Zhang
- C Programming Boot Camp by Paul Gribble
- devdoc - c
- C Programming Language Basics by Chua Hock-Chuan
- stackoverflow c doc
Źródła
[edytuj]- ↑ stackoverflow :compiling-objective-c-on-ubuntu-using-gcc
- ↑ askubuntu question: difference-between-libasan-packes-libasan0-libasan2-libasan3-etc
- ↑ stackoverflow question: graphics-library-in-c
- ↑ moderncprogramming: how-can-you-draw-shapes-in-c-easily
- ↑ stackoverflow question: reading-an-image-file-in-c
- ↑ libc backtrace
- ↑ libunwind
- ↑ Postmortem Debugging by Stefan Wörthmüller
- ↑ nanolat by Kangmo Kim
- ↑ Catching Exceptions and Printing Stack Traces for C on Windows, Linux, & Mac BY: JOB VRANISH
- ↑ Stack unwinding (stack trace) with GCC
- ↑ Programowanie w języku C - Artur Pyszczuk